๋ฌธ์
Given an array of integers nums sorted in non - decreasing order, find the starting and ending position of a given target value.
If target is not found in the array, return [-1, -1].
You must write an algorithm with O(log n) runtime complexity.
Example 1:
Input: nums = [5,7,7,8,8,10], target = 8
Output: [3,4]
Example 2:
Input: nums = [5,7,7,8,8,10], target = 6
Output: [-1,-1]
Example 3:
Input: nums = [], target = 0
Output: [-1,-1]
Constraints:
- 0 <= nums.length <= 105
- -109 <= nums[i] <= 109
- nums is a non-decreasing array.
- -109 <= target <= 109
๋น๋ด๋ฆผ์ฐจ์์ผ๋ก ์ ๋ ฌ๋์ด์๋ ์ซ์ ๋ฐฐ์ด์ ์ฃผ๊ณ ์ฃผ์ด์ง ํ์ผ ๋ฐธ๋ฅ๋ฅผ ์ฐพ์์ ์ฒ์ ํฌ์ง์ ๊ณผ ๋ ํฌ์ง์ ์ ๋ฐํํ์์ค
๋ง์ฝ์ ํ์ผ์ด ์๋ค๋ฉด [-1, -1]์ ๋ฐํํ์์ค
๋น ์ค ์ด์ง๊ฒ์์ ์๊ฐ ๋ณต์ก๋๋ก ์ฐ์์ค- ?
*๋น๋ด๋ฆผ์ฐจ์ ๋ป
๋ด๋ฆผ์ฐจ์์ ์ ์ ๊ฐ์ํ๋ ์์ด์ด๊ณ ๋น๋ด๋ฆผ์ฐจ์์ ์ ์ ์ฆ๊ฐํ๋ ์์ด์ ๋๋ค. ์คํ๋ ค ๋น๋ด๋ฆผ์ฐจ์์ ์ค๋ฆ์ฐจ์๊ณผ ๋น์ทํ๋ฐ, ์ฐจ์ด์ ์ ์ค๋ฆ์ฐจ์์ ์ธ์ ํ ๋ ์๊ฐ ๊ฐ์ ์๋ ์๋์ง ์ฌ๋ถ๋ฅผ ๋ช ํํ๊ฒ ์๋ ค์ฃผ์ง ์์ง๋ง ๋น๋ด๋ฆผ์ฐจ์์ ๊ฐ์ ์๋ ์์์ ๋ช ํํ ํด์ค ๊ฒ์ด๋ผ๊ณ ๋ณผ ์ ์์ต๋๋ค.
ํด๊ฒฐ ์ฝ๋
/**
* @param {number[]} nums
* @param {number} target
* @return {number[]}
*/
var searchRange = function(nums, target) {
let temp =[]
// ์ฃผ์ด์ง ์ธ์ง์ ๊ธธ์ด๊ฐ 0์ผ ๋
if(nums.length == 0) {
return [-1, -1]
// ์ธ์์ ๊ธธ์ด๊ฐ 0์ด ์๋ ๋
}else{
//์ ํ๊ฒ์์ผ๋ก ๋๋ฉด์ ํด๋น target ์ธ๋ฑ์ค ์ฐพ์์ array์ ๋ฃ์ด์ฃผ๊ธฐ
for(let i=0; i<nums.length; i++ ){
if(nums[i] === target){
temp.push(i)
}
}
// ๋ง์ฝ์ ๋ง๋๊ฒ ์์ด์ ์ธ๋ฑ์ค๊ฐ ์ ๋ค์ด๊ฐ๋ค๋ฉด
if(temp.length == 0){
temp= [-1, -1]
// ๋ค์ด๊ฐ ์ธ๋ฑ์ค๊ฐ ์๋ค๋ฉด ์ ์ผ ์ฒ์๊บผ๋ ์ ์ผ ๋๋ถ๋ถ๋ง ์ฐพ์์ ๋ฃ์ด์ฃผ๊ธฐ
}else{
temp = [temp[0], temp[temp.length-1]]
}
}
return temp
};
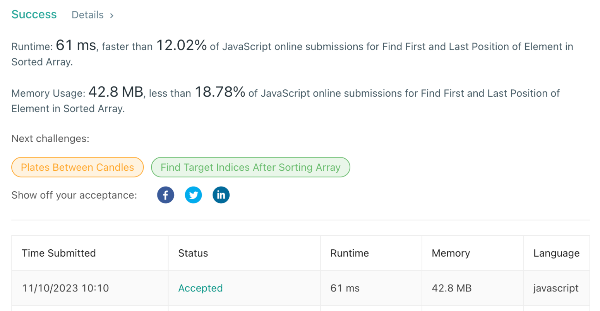
'๊ฐ๋ฐ > ๐ฌ ์ฝ๋ฉํ ์คํธ' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[leetCode] 2215. Find the Difference of Two Arrays (1) | 2024.01.30 |
---|---|
[leetCode/EASY] 1431. Kids With the Greatest Number of Candies (0) | 2024.01.17 |
[leetCode/์ฝํ ๊ณต๋ถ] 2721. Execute Asynchronous Functions in Parallel (0) | 2023.08.22 |
[leeCode/์ฝํ ๊ณต๋ถ] 2625. Flatten Deeply Nested Array (0) | 2023.08.21 |
[์๊ณ ๋ฆฌ์ฆ] .val ๊ณผ .next ์ ๋ป์? (0) | 2022.12.30 |